In this technical deep dive, we'll explore how to build a Financial Facts Checker using
OpenAI's Agent SDK. For this, we will use Python and
FastAPI as our base Setup as we want to build an API. This FastAPI-based API is capable of validating financial statements (i.e. from your favorite financial influencer) using AI agents with built-in guardrails and web search capabilities.
Introduction#
The
OpenAI Agents SDK is a recent release from OpenAI offering a straightforward way of implementing AI agents. With easy to use capabilities such as Tool calling, Guardrails, Handoffs it's a wonderful SDK for building and interacting with AI in an "agentic" way.
Project Overview#
What do we need in order to build such a project? For this, we will do the following steps:
- Set up AI agents with specific roles and responsibilities
- Implement input guardrails for validation
- Use web search capabilities for fact-checking
- Create a REST API interface for the agent system
Technical Architecture#
Project Structure#
The project has the following file structure. This enables us to easily extend this API in order to be flexible for further use cases:
1agents_sdk/
2├── app/
3│ ├── __init__.py
4│ ├── models.py # Pydantic models
5│ ├── routes.py # API endpoints
6│ └── agents/ # Agent definitions
7│ ├── fincial_checker_agent.py
8│ └── guardrail_agent.py
9├── main.py
10└── requirements.txt
Key Components#
The project has two main components:
-
Agent System: Two specialized agents working together
- Financial Facts Checker Agent: Main agent for validating financial information
- Guardrail Agent: Pre-check agent ensuring inputs are finance-related to avoid unwanted user input hitting our main agent. This is especially helpful when dealing with a public-facing Agent setup.
-
FastAPI Backend: RESTful API interface
- Input validation using
pydantic
models
- Async endpoint handling
- Structured response formatting
Deep Dive into the Implementation#
The next section will provide a technical deep dive as well as an explanation of the individual steps:
1. Agent Definitions#
Let's start with the guardrail agent, which acts as our first line of defense.
Guardrails enable you to do checks and validation on user input (or agent output):
python
1@input_guardrail
2async def financial_content_guardrail(ctx: RunContextWrapper, agent: Agent, input: str | list[TResponseInputItem]) -> GuardrailFunctionOutput:
3 result = await Runner.run(guardrail_agent, input, context=ctx.context)
4 return GuardrailFunctionOutput(
5 output_info=result.final_output,
6 tripwire_triggered=not result.final_output.is_financial_content,
7 )
8
9guardrail_agent = Agent(
10 name="Guardrail check",
11 instructions="Check if the user is asking you a request that is related to financial content.",
12 output_type=FinancialContentGuardrail,
13)
The guardrail agent uses the @input_guardrail
decorator to validate incoming requests. It ensures that only finance-related queries are processed.
The main financial facts checker agent is more complex. This is a general
Agent capable of doing what was described to it in the instruction section:
python
1financial_facts_checker_agent = Agent(
2 name="Financial Facts Checker Agent",
3 handoff_description="A helpful agent that can answer whether the provided content is correct financial information.",
4 input_guardrails=[financial_content_guardrail],
5 instructions="""
6 [Instructions for checking financial facts...]
7 """,
8 model="gpt-4",
9 tools=[WebSearchTool()],
10 output_type=FinancialCheckerOutput
11)
The key features of this agent are:
- Uses GPT-4 as the base model
- Implements web search capabilities (A tool provided by OpenAI' Agent SDK to execute Web Search)
- Has structured output typing (see OutputType here)
- Includes input guardrails
2. API Implementation#
The API layer is built with
FastAPI and uses
Pydantic models for request/response validation:
python
1class FinancialContentRequest(BaseModel):
2 content: str
3
4class FinancialContentResponse(BaseModel):
5 is_correct: bool
6 reasoning: str
The main endpoint implementation:
python
1@router.post("/check-financial-content", response_model=FinancialContentResponse)
2async def check_financial_content(request: FinancialContentRequest):
3 input_items = [{"content": request.content, "role": "user"}]
4 result = await Runner.run(financial_facts_checker_agent, input_items)
5 return FinancialContentResponse(
6 is_correct=result.final_output.is_correct,
7 reasoning=result.final_output.reasoning
8 )
This is the main logic pretty much calling the user input and starting an execution using the
Runner.run method. This starts the process chain with the user provided input. Attention: For a production implementation it would be beneficial to add additional input checks to avoid direct user input.
Working with OpenAI's Agent SDK#
OpenAI's Agent SDK provides the following key concepts:
Key Concepts#
-
- Agents are defined with specific instructions and capabilities
- They can use tools (like web search)
- Output is strictly typed using Pydantic models
-
- Prevent misuse of agents
- Validate input before processing
- Can chain multiple guardrails
-
- Handles agent execution
- Manages context and state
- Provides structured output
Best Practices#
Here are our best practices for implementing an AI agent API:
-
Type Safety
- Use Pydantic models for all inputs/outputs
- Define clear response structures
- Validate all external inputs
-
Error Handling
- Implement proper exception handling
- Use HTTP status codes appropriately
- Provide meaningful error messages
-
Agent Design
- Keep instructions clear and specific
- Use appropriate model versions
- Implement necessary tools
Using the API#
In order to use the API, please run the following commands:
bash
1# Start the server
2python -m main
3
4# Make a request
5curl -X POST "http://localhost:8000/check-financial-content" \
6 -H "Content-Type: application/json" \
7 -d '{
8 "content": "An ETF is the same thing as an individual Stock"
9 }'
The API will return a structured response:
json
1{
2 "is_correct": false,
3 "reasoning": "This statement is incorrect. While both ETFs and individual stocks are investment vehicles traded on exchanges, they are fundamentally different..."
4}
Additionally, for easier testing,
FastAPI provides a
swagger
endpoint (
/docs
) out of the box, which can also be used for testing:
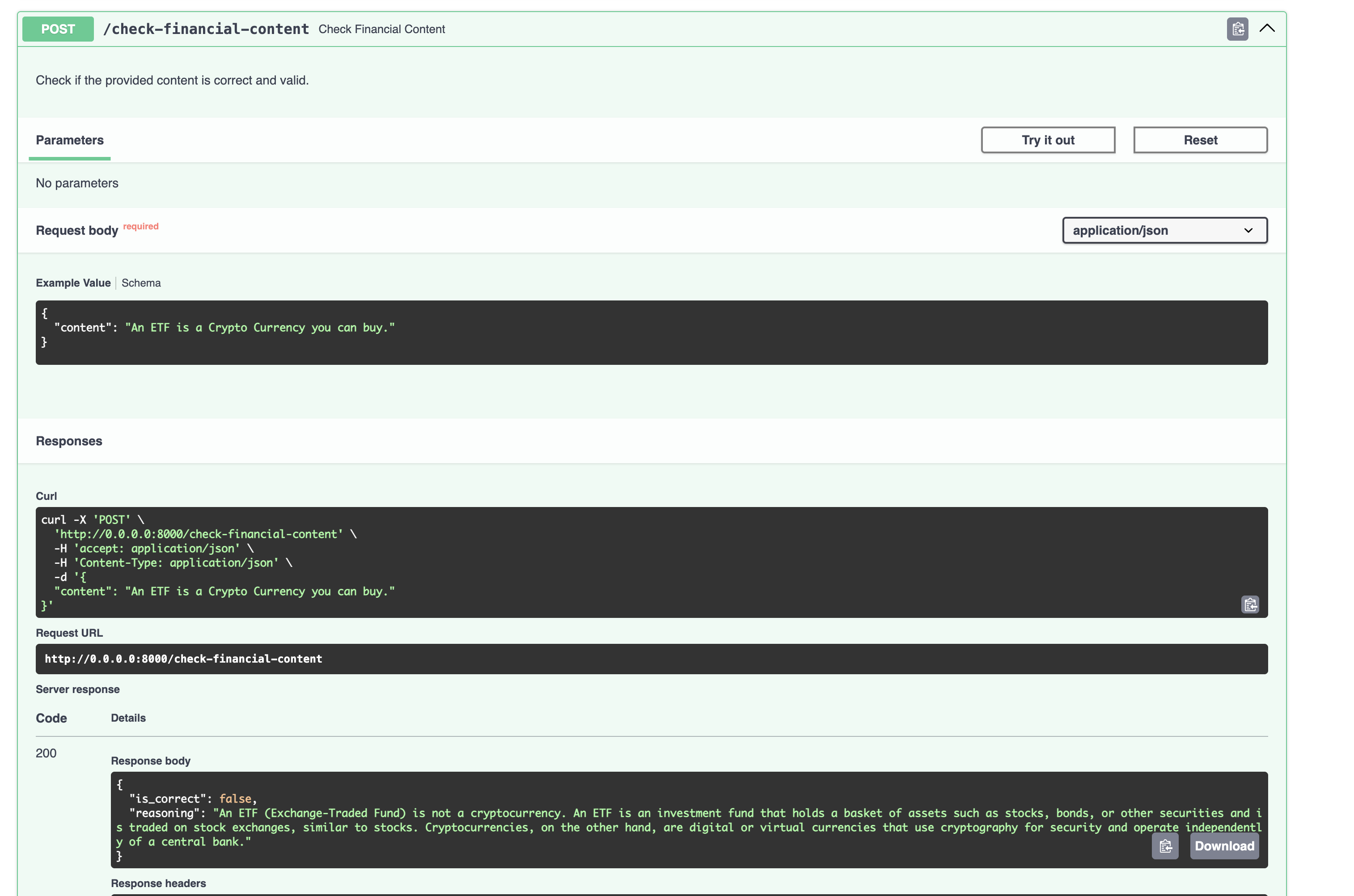
Testing the API using Swagger (/docs)
Conclusion#
OpenAI's Agent SDK provides a powerful framework for building AI-powered applications. By combining it with
FastAPI and implementing proper guardrails, we can create robust, production-ready services that leverage AI capabilities safely and effectively.
The Financial Facts Checker demonstrates how to:
- Structure an agent-based application
- Implement proper validation and guardrails
- Create a user-friendly API interface
- Handle complex AI interactions in a production environment
This implementation serves as a template for building similar AI-powered services using OpenAI's Agent SDK, showing how to properly structure the code, handle errors, and create a maintainable system. You can find the entire example project
here.
For organizations looking to implement their own AI agents, our
agency specializes in developing custom AI agents and integrations tailored to specific business needs. We help bridge the gap between your organization’s knowledge repositories and the AI systems your users interact with.
Note: The OpenAI Agents SDK is a rapidly evolving technology. The information in this article is current as of March 2025. For the most up-to-date information, please refer to the official specification and documentation.